Overview
Why JavaScript?
In this article we focus on client-side cookies; Cookies generated by, and stored in your browser.
The most commonly used (and readily available) scripting language for that, would be JavaScript – by the best of my knowledge all modern browsers support this, and it does not require the user to install plugins like Flash and such.
Ad Blocking Detected Please consider disabling your ad blocker for our website.
We rely on these ads to be able to run our website.
You can of course support us in other ways (see Support Us on the left).
What are Cookies?
Cookies are in essence a means to store data locally (client side), in the browser of the user that is visiting your webpage. You could see cookie data als a small text that contains the information you’d like to store, which can be accessed again at a later time.
For example the users preferences or information like; Shopping cart content, Last viewed items, or previous page, How many rows to show in a list per page, Which page you’re on when paginating a long list, Preferred name, Preferred font size, etc.
For example I use cookies in Tweaking4All for remembering where the user was last in the menu tree (the menu on the left), and to remember the screen resolution and responsive design preferences (desktop versus mobile view). In the meanwhile it is also being used for logging in and some of the plugins my website uses.
Cookies have gotten a bad rep because of advertising firms using it to “store” your surfing behavior, so they can target their advertisements better. You can argue if this is a bad thing or not – but do keep in mind that these advertisements contribute to paying for free information, and does it really matter that an ad that is being displayed better matches your needs?
As usual, with most of these topics, we tend to either ignore the issue or go way overboard with regulations (hello retarded EU Cookie law, and hello retarded EU law on maximum power on vacuum cleaners – in all honesty, i think we have bigger problems to deal with). Anyhoo, I better not go on a rant on these topics.
Bottom line:
Cookies still work, and will keep working in the future, and certain politicians should start looking for a real job.
Those users who disable cookies will find that most websites will not work as expected, so I’m guessing that the majority of users still allows cookies.
Please note that there are several kind-a alternatives to cookies, server-side included, but they all have their own downsides and complexity.
Some Cookie Lingo
There are several cookie types and expressions, here a very short list:
Session Cookie, is a cookie that gets deleted when the user closes the browser so it’s of a temporary nature.
Persistent Cookie on the other hand is a cookie that has an expiration date/time. It’s information remains available, even after closing a browser, as long as it has not reached it’s expiration date or time.
A Secure Cookie can only be transmitted over an encrypted connection (for example over HTTPS) and cannot be transmitted over unencrypted connections (HTTP). Theoretically this makes a cookie more secure.
Then there is the so called HTTPOnly Cookie, which can only be accessed by the particular server. So this is a local cookie that only can be accessed if the web-server needs it and NOT by JavaScript. This makes a cookie more secure for example for session information. Your JavaScript however cannot read it (if the browser implements HTTPOnly correctly of course).
When talking about a Cookie Domain, we mean that we assigned a specific domain to a cookie. Code from other domains will not see these cookies.
The SameSite Cookie can only be accessed from the same domain it has been created with. You could compare this with setting a fixed domain for a cookie.
Let’s say the user went to Google.com and the Google page stored the search phrase as a cookie.
In case of a SameSite cookie, the cookie would originate/belong to the google.com domain.
Now when the user opens a webpage from for example Tweaking4All.com, the code in this newly opened webpage would not be able to access the SameSite cookie – thus keeping the stored data safer.
For a cookie you can set a specific path as well, the so called Cookie Path. For example a cookie value that would only be accessible when the web-page is in a given path (and domain), making it’s “range” even tighter and more secure.
Most browsers also block so called Super Cookies.
A Super Cookie can be seen as a SameSite Cookie or a Cookie with a defined domain, but instead of using a regular domain (google.com) it uses the top-level domain (for example everything that ends with .com). So a SuperCookie can be accessed from any other .com domain for example, which is of course a major security concern.
Finally there is the Zombie Cookie or Ever Cookie – which keep regenerating themselves even after you delete them. When the cookie’s absence is detected, the cookie is recreated using the data stored in these locations. This appears to happen with certain HTML5 and Flash situations that I’m not familiar with.
Ad Blocking Detected Please consider disabling your ad blocker for our website.
We rely on these ads to be able to run our website.
You can of course support us in other ways (see Support Us on the left).
Playing with JavaScript, and Looking in the Cookie Jar
Before we start poking around in the JavaScript code, a few important tips that make getting started easier.
Playing with JavaScript
There are a few ways to play with JavaScript. I have used the 3 listed below on different occasions.
Recommended – JSFiddle
The easiest way is by just running your JavaScript in JSFiddle, which is what I would recommend to start with.
Go to the JSFiddle website, enter the JavaScript code in the “JavaScript” box (where you see “alert(‘hello World’);” in the image below) and click the “Run” button at the top.
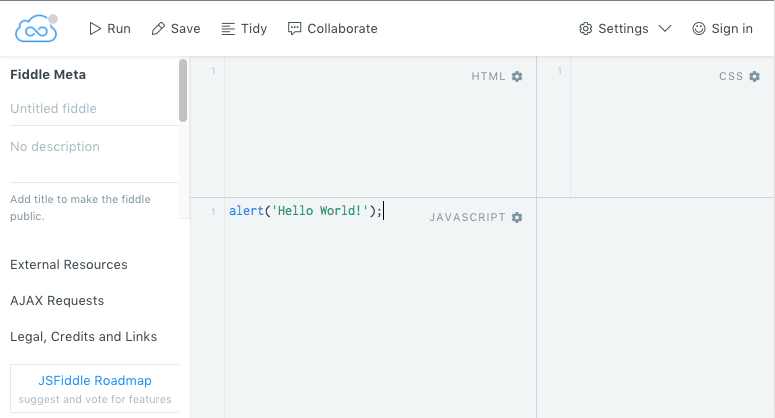
JSFiddle – Awesome free online to test JavaScript
Old School – Just a file on your computer
What used to be the easiest way, was by simply creating a HTML (plain text) file with NotePad on your desktop, but with modern browsers that can come with unexpected complications.
When playing with JavaScript code in a local file (for example store on your desktop), you will find that not all browsers will handle this correctly. This is done for security reasons (would be nice if the user could override this – yes Mr. Google, I’m talking to you!).
Normal webpages are opened from a web-server using http:// or https://.
A file on your desktop however, will be opened with file:// and this is where certain browser will block certain activities like accessing cookies.
So far (on my Mac) I have noticed that Safari is playing nice (version 10.1.1 in macOS Sierra 10.12.5), but Google Chrome is not (version 59.x). Tricks for Google Chrome to enable this in do not always seem to work or appear at least unreliable and cumbersome.
Fancy – Your own web-server
A little harder to do would be using your own web-server, which is not super hard to do, but a maybe more work than one would want to do for some simple playing with JavaScript.
A web-server does not require a magically server room, like we see them in the movies with rows and rows beautiful black server racks, with blinking lights and nicely organized or even invisible cables all over the place.
You can simply install Apache (the web-server software) or even better: use one of those AMP (Apache, MySQL, PHP) bundles like MAMP, XAMPP, WAMPServer, etc. and run your “web-server” straight from your desktop or laptop. Most of these are free and setup can be straight forward.
Also note that most decent NAS devices, like QNAP and Synology, come with a web-server already installed.
Spying on Cookies – or: How to peek in the cookie jar
Now under normal circumstances, we (the user) will not see what cookies are being stored by a website.
We could of course use the JavaScript function Alert('Some text here');
to display the values of our cookies, or use functions like document.write
to insert it in the HTML content. But this can be done easier since most modern browsers do come with some sorts of developer tools to view these cookies and their values (and a whole bundle of other things).
As an example, Google Chrome (most modern browsers offer such a feature).
Open an webpage you like and right click somewhere in the content. A menu pops up and one of the options at the bottom says “Inspect” – click “Inspect” option a some interesting stuff appears. Feel free to try this even on this page.
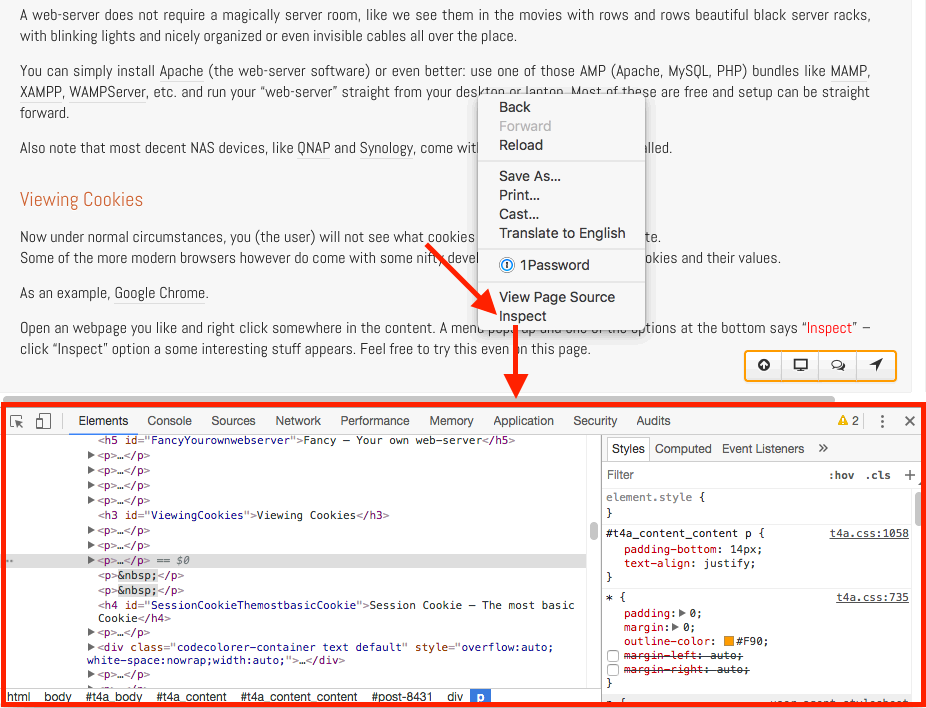
Google Chrome – Web Developer view
In case the view does not open at the bottom, but for example on the side, then you can change that by clicking the 3-dots button and select the view you prefer.
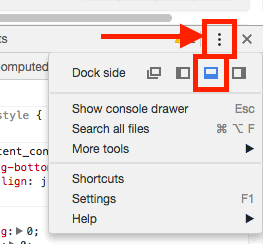
Google Chrome – Change view
To see the store cookie values, click “Applications” on top, and on the left side under “Cookies” choose the link of the webpage you’re looking at. All cookies for this domain will now be shown as a Name-Value pair and additional options like Domain, Path, Expiration etc.
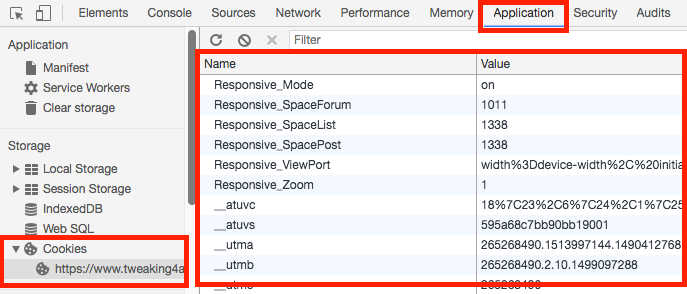
Google Chrome – View Cookies
Just above the grid, on the left, just above the “Name” column, you will also see 3 buttons which you will use frequently: “Refresh“, “Delete All Cookies” and “Delete Selected Cookie“.
Clear and Refresh Cookies when playing with JavaScript code!
Before testing a particular JavaScript, with this web developer window open, please consider clicking the “Delete All Cookies” button and after running your script click “Refresh” to see the effect. Otherwise a cookie might come through which might have been a leftover from previous tests, or the cookie list might no longer reflect the current values.
What do we find in a Cookie?
Browser settings: Enable cookies
In the following examples and code, we assume you have cookies enabled in your browser.
Now that we can play with JavaScript and know how to find out what the cookie values are, on to the “real” stuff …
So … a cookie is stored as plain text, and it’s most elementary form is a value pair: name = value,
Each of these pairs can have additional properties like for example expiration date/time, wether or not it is secure, etc.
To do all this, we use the JavaScript “document.cookie” property. Yes, it is a property and not a function. See it as a string variable that holds text – and the text is just a bunch of sequences of name = value separated by semi colons and therefor holds all cookies we have access to.
For example “username=Hans; location=Houston; weather=Sunny; beer=Low;” …
Properties, like expiration data and such cannot be read! Only written!
Creating a cookie is simply the same as defining a value pair – which automatically adds this pair to the string called “document.cookie“.
Changing the value in a cookie is done by defining a value pair with the same “name” but holding a different value, which then automatically replaces the old value.
Deleting a cookie is done by simply letting them expire by setting the expiration date in the past.
So to display the value of all our cookies (that we get access to) would be done like so:
1 2 3 4 5 6 7 8 9
| <html>
<body>
Hello there!</br>
<script language="javascript">
var myCookie = document.cookie; alert(myCookie); </script>
</body>
</html> |
The variable “myCookie” gets a copy of the text stored in “document.cookie”. The “alert()” function then displays is as a popup dialog.
Obviously, when you run this right now, it would display an empty message, since we have not stored a cookie yet.
Also keep in mind that I create a full (minimalistic) webpage with this code, HTML included, which would be the way to do it if you would want to test this as a file on your desktop (name it something like test.html) or on your web-server if you have one.
Beyond this point I will only show the JavaScript code (line 5 and 6 in this example), since that’s the only part that would change and it is the part we simply paste in the JavaScript box at JSFiddle (in JSFiddle you do not need to enter anything else in the window to make it work).
Ad Blocking Detected Please consider disabling your ad blocker for our website.
We rely on these ads to be able to run our website.
You can of course support us in other ways (see Support Us on the left).
Setting Different Cookie kinds in JavaScript
In this section I’ll show you how to create different kind of cookies, in JavaScript.
Session Cookie – My First Cookie
We will start with the most basic cookie: the Session cookie. This would be simplest cookie you can create and is referred to as a “Session Cookie” since it does not live outside of a session, so it gets deleted automatically when you close the browser.
Information of a cookie is stored in value pairs, and it’s most simple form would look something like this:
Storing sensitive information like usernames and such, in a cookie, is not really a BAD idea – here it just serves as an example.
Below the JavaScript code which you can paste in JSFiddle;
document.cookie = "username=Hans";
Obviously, we didn’t see anything exciting happen, so use the web developer tools of your browser to see that the cookie has actually been set. You can use the “Inspect” option from Google Chrome on the JSFiddle page as well.
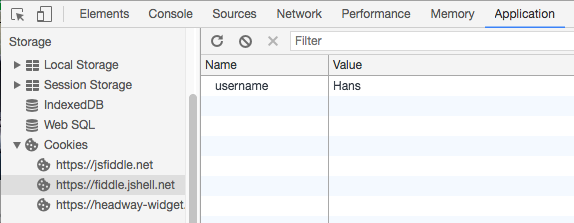
My First Cookie!
If you’d prefer to not use the browsers web developer tools, you could also use an alert, which would look something like this:
1 2
| document.cookie = "username=Hans";
alert(document.cookie); |
The result should be a popup, showing something like this:
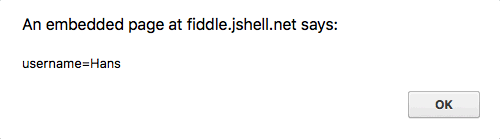
My First Cookie – Alert
Alrighty then … we have the basics kind-a under control, so now time to do some more advanced things by adding options. These options are stored as value pairs as well, in the same document.cookie property. Let’s go through them one at a time …
Persistent Cookie – A Cookie that survives closing the browser
As mentioned before, we can make a cookie persistent by defining it’s expiration date and time, which would look like something like the example below:
username=Hans; Expires=Sun, 22 Oct 2017 08:00:00 UTC;
This will also be used to delete a cookie, by setting the expiration date to a date in the past – your browser will remove the cookie based on that alone.
The date format for cookies must be in a UTC/GMT format (i.g. Sun, 22 Oct 2017 08:00:00 UTC), so correct for your timezone if needed.
UTC versus GMT: both indicate the same time, however: UTC is a time standard, whereas GMT is a timezone. UTC is preferred.
OK,… ready for an example – here it can be very helpful to look at the earlier mentioned “Inspect” (web developer) tools, since it lists these properties as well. Our JavaScript will look something like this (remember to change the date here if you’re trying this after Oct 22nd 2017 8 AM UTC):
1 2
| document.cookie = "username=Hans; Expires=Sun, 22 Oct 2017 08:00:00 UTC;";
alert(document.cookie); |
And when inspecting the cookies with Google Chrome (for example), we will see something like this:

Cookie Details
Here we can see the name, value, domain, path, expiration date/time, size (number of characters of name + value), HTTPOnly, Secure and SameSite. The alert on the other hand only shows “username=Hans”.
A few things to note here, and the alert() message kind-a points in that direction as well this:
- document.cookie only holds the name-value pairs that we are allowed to see,
- properties of a value pair (like expiration) is not returned to use when we read document.cookie.
The fact that we can see this info in the web developer tool, is because these tools are part of your browser and allow looking into the inner workings – which is great for developers and for us while we are learning more about cookies.
Secure Cookie – Which only works when HTTPS is being used
Making a cookie secure is pretty easy as well, we simply add the option “Secure”.
This one can be a tricky one to test with a local file or your own web-server, since this one would only work over a HTTPS connection which typically is not the case. JSFiddle on the other hand uses HTTPS so it will work there;
1 2
| document.cookie = "username=Hans; Secure;";
alert(document.cookie); |
The “Inspect” option in Google Chrome, might require you to click the refresh button in the web developer tools (as indicated before). You will now see a checkmark in the “secure” column as well.
HTTPOnly Cookie – The Cookie that can only be accessed by the web-server
The same goes for making a HTTPOnly cookie, we just add “HttpCookie” – before doing so, you might want to delete all cookies in the web developer tools of your browser.
1 2
| document.cookie = "username=Hans; HttpOnly;";
alert(document.cookie); |
Now refreshing the cookies in the web developer tools should not show a cookie, and neither does the “alert()” … which is correct. The cookie exists, but we just are not allowed to access it with JavaScript.
SameSite Cookie – A Cookie only for this website
SameSite cookies, if supported by the browser, knows two variants: strict and lax.
Here strict is the preferred setting, since it closes down all cross domain access.
1 2
| document.cookie = "username=Hans; SameSite = strict;";
alert(document.cookie); |
When you want to allow “some” cross domain access:
1 2
| document.cookie = "username=Hans; SameSite = lax;";
alert(document.cookie); |
This again is a tricky one, since it might only show something with your local web-server. A file or JSFiddle might not show anything in the “alert()”, but the web developer tools will show that the cookie actually does exist.
Cookie Domain – For Cookies that are only for a specific domain
We can define a domain and path in which a cookie can be accessed.
To limit a cookie to a certain domain, you can try the following two examples. The first one will use a random domain, example.com. The second example will use the domain jshell.net – which is the domain used by JSFiddle.
Note : when entering “example.com” as the domain, the cookie can only be accessed from that exact domain, but not from sub domains. When adding a period in front of the domain, for example “.example.com” then the cookie can be accessed from sub domains as well (ie. forum.example.com, email.example.com, www.example.com, etc).
1 2
| document.cookie = "username=Hans; domain = .example.com;";
alert(document.cookie); |
You will notice that there is no cookie and the alert is not showing anything either – which again is correct since we are not allowed access to these cookies, since we are working in the wrong domain (not example.com).
1 2
| document.cookie = "username=Hans; domain = .jshell.net;";
alert(document.cookie); |
This second example WILL generate a cookie that is visible and the alert will show the cookie as well (when testing this in JSFiddle, since JSFiddle runs in the jshell.net domain).
Cookie Domain and Path – For Cookies that are only for a specific path and domain
We can narrow this down by adding a path, which would only allow access to this cookie if we are in the right domain and path (if Domain is not defined, the current domain will be used).
The following example the following tells the browser to only access this cookie when we are in the path /secretdata (on the current domain). So if we would be working on the server with domain example.com, this would work for pages in for example http://example.com/secretdata and it’s sub paths like for example http://example.com/secretdata/morestuff. Obviously this is a little trickier to test – and you might not need this ever.
It is recommended to add the “Path” to your cookies so the browser will not get confused too much. By default the cookie will be relative to the page path you’re working with. But if you want to access the cookie from another page on your website, then the cookie might actually not be accessible. If the cookie should be readable throughout your entire dome then always add: “Path=/;“.
1 2
| document.cookie = "username=Hans; path = /secretdata;";
alert(document.cookie); |
To illustrate how things get confusing, try the following code. The first “username” cookie will be relative to this page, the second “username” relative to the entire site. Since one was defined without path (relative to this page) and one with path (relative to the website), suddenly the browser has 2 cookies with the same name – which obviously might result in unexpected behavior when trying to read the cookie.
1 2 3 4 5 6 7
| document.cookie="username=Hans";
alert(document.cookie);
document.cookie="username=John; Path=/;";
alert(document.cookie);
document.cookie="username=Banana;";
alert(document.cookie); |
In the example above: first we create the cookie username=Hans relative to this page and the alert confirms this.
The next cookie however is defined as a new (!) cookies username=John with Path=\ (enitre domain).
The alert now actually shows 2 cookies with the same name: username=Hans; username=John. So which one do we use in our code?
In the 3rd part we change the value of one of the page relative cookies, for example because we forgot to add the path, and guess what … things become messy real quick.
Here you can see how important it can be to properly define the path.
Combining options – A Cookie jar of options …
All these options can be combined in a single line as well, separated by semi colons, even when it might make little or no sense:
1 2
| document.cookie = "username=Hans;Path=/; Domain=email.example.com; Expires=Sun, 22 Oct 2017 08:00:00 UTC; Secure; HttpOnly; SameSite = strict;";
alert(document.cookie); |
So this cookie holds the username=Hans value pair, can be used until Sunday October 22nd, 8 AM UTC (GMT), and only be accessed in the email.example.com domain with the path /secretdata, cross domain use is prohibited (SameSite), HTTPS is required (secure) and it’s a HTTPOnly cookie (which can only be read by the server).
Updating a Cookie with JavaScript
Updating a cookie works the exact same way as creating a cookie. We simply create the cookie again, with the same name, and use a different value. With this update you can also right away change certain properties like “Secure”, Expiration date and time etc. But … you can only update those you have access to, so if you created for example a HttpOnly cookie, and you’d like to update that to a normal cookie, then actually two cookies will exist with the same name – one visible to you and your JavaScript, and one only the server can see.
A quick example:
1 2 3 4 5 6 7 8 9 10 11
| document.cookie="username=Hans; Path=/";
alert(document.cookie);
document.cookie="username=Martha; Path=/";
alert(document.cookie);
document.cookie="username=John; Path=/";
alert(document.cookie);
document.cookie="username=Diana; Path=/";
alert(document.cookie); |
This example will keep changing the names store in the “name” cookie – as the alerts will confirm. See how we use the “Path=/” consistently? (now that we know how bad things can become)
Ad Blocking Detected Please consider disabling your ad blocker for our website.
We rely on these ads to be able to run our website.
You can of course support us in other ways (see Support Us on the left).
Deleting a Cookie with JavaScript
I have already mentioned this one, and now that we know that we can update a cookie [that we have access to], we can delete a cookie by simply setting the expiration date to a date in the past. Jan 1st 1970 seems to be the most popular date for this purpose:
Thu, 01 Jan 1970 00:00:01 UTC
This would be one of the closest numbers to date and time being zero on quite a few systems, this is actually the date and time where the clock starts counting – point zero (time is stored as a number, expressed in days, starting January 1st, 1970).
I have seen it happen where this kind of deleting actually results in a Persistent Cookie just becoming a Session Cookie – so the cookie is not really gone until we close the browser. Therefor it is always a good idea to assign the cookie no value (empty string).
Below some examples illustrating the behavior in your browser. I did experience some inconsistency with the second delete, where we do set a value. Therefor I would recommend the last example where we set the value to nothing – just to make sure.
Here you see again how important setting the path can be.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| // Set cookie (website)
document.cookie="username=Hans; Path=/;";
alert('Cookie set: '+document.cookie);
// Forgot path, cookie did not get deleted:
document.cookie="username=Banana; Expires=Thu, 1 Jan 1970 00:00:01 UTC;";
alert('Cookie still set: '+document.cookie);
// Expires but new value - I did get inconsistent results here
document.cookie="username=Bram; Path=/; Expires=Thu, 1 Jan 1970 00:00:01 UTC;";
alert('Cookie might be set or not: '+document.cookie);
// Set cookie again:
document.cookie="username=Hans; Path=/;";
alert('Cookie set: '+document.cookie);
// recommended:
document.cookie="username=; Path=/; Expires=Thu, 1 Jan 1970 00:00:01 UTC;";
alert('Cookie expires and cleared: '+document.cookie); |
Some Practical JavaScript functions
As we have seen, handling cookies is not hard, but sometimes it’s nice to have some tools to make it just a little easier.
Below a few tools, that might get you started.
getCookie() – Reading Specific a Cookie in JavaScript
In JavaScript we can use document.cookie again to read cookies as well. But here is where it becomes a little messy.
Let’s look at this example:
1 2 3
| <script language="javascript">
var myCookie = document.cookie;
</script> |
The variable “myCookie” will contain all text that you’re allowed to pull in for this given domain/path/etc. which results in listing all know value pairs that match these criteria. So if we defined 4 cookies, that match the criteria, then the result would contain all the value pairs! Something like:
cookie1=value1; cookie2=value2; cookie3=value3; cookie4=value4
As you can see, we get all cookies that we are allowed to see back as an answer – not very practical when you’re looking for just one value pair isn’t it? So this is where we use the following simple function – I’ll show it with comments so you know what it’s doing.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| // The getCookie function
function getCookie(cookiename) {
var name = cookiename + "=";
// Decode URI to normal if needed and split into an array of cookies
var arrayOfCookies = decodeURIComponent(document.cookie).split(';');
// examine all elements in the cookie array (list)
for (var i = 0; i < arrayOfCookies.length; i++) {
// Get the next cookie from the array
var aCookie = arrayOfCookies[i];
// Skip prefix spaces
while (aCookie.charAt(0) == ' ') {
aCookie = aCookie.substring(1);
}
// if the cookie name is found, return the value part of the string
if (aCookie.indexOf(name) == 0) {
return aCookie.substring(name.length, aCookie.length);
}
}
// return nothing if we didn't find the cookie name
return "";
} |
The workings of the function is explained in the code comments.
The short version: grab the document.cookie, and break it up in an array (a list of strings) with one item (line) per cookie. Now for each line we remove redundant prefix spaces, and after that we see for each the line, one at a time, is a match for the cookie we are looking for. As soon as we find a match we return the value found in that particular string. If we end up finding nothing, we will return an empty string;
Now I did cleanup this function and added some example cookies so you get an idea how this works, and right away have a compact function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| // Set a few cookies (website)
document.cookie = "username1=Hans1; Path=/;";
document.cookie = "username2=Hans2; Path=/;";
document.cookie = "username3=Hans3; Path=/;";
alert('Cookies set: ' + document.cookie);
alert('Found this value for "username3": ' + getCookie('username3'));
// the getCookie function
function getCookie(cookiename) {
var name = cookiename + "=";
var arrayOfCookies = decodeURIComponent(document.cookie).split(';');
for (var i = 0; i < arrayOfCookies.length; i++) {
var aCookie = arrayOfCookies[i];
while (aCookie.charAt(0) == ' ') { aCookie = aCookie.substring(1); }
if (aCookie.indexOf(name) == 0) {
return aCookie.substring(name.length, aCookie.length);
}
}
return "";
} |
In case you really want to optimize things, you could use a JavaScript Compressor – it is sometimes amazing what this can do (for example this one: JSCompressor). Compressing JavaScript comes with a downside though: the result works but is sometimes hard to read:
1
| function getCookie(e){for(var n=e+"=",t=decodeURIComponent(document.cookie).split(";"),o=0;o<t.length;o++){for(var r=t[o];" "==r.charAt(0);)r=r.substring(1);if(0==r.indexOf(n))return r.substring(n.length,r.length)}return""} |
setCookie() – Creating and/or updating a Cookie
The function I’m using here is limited to the basics like defining the value pair, and setting an expiration date. The path is fixed to Path=\.
Expanding this function with “Secure”, “HttpOnly” and “SameSite” is not that hard – especially when we make it a fixed part.
Note that with setting a Cookie I mean either update an existing cookie, or create it when it does not yet exist.
Additionally I’ve added 3 possible scenario’s for the days until the new cookie expires;
Value |
Effect |
< 0 |
Delete the Cookie by setting the expiration date to “Thu, 1 Jan 1970 00:00:01 UTC” |
0 |
Do not set expiration date (make it a session cookie) |
> 0 |
Set the cookie to expire in x number of days (persistent cookie) |
1 2 3 4 5 6 7 8 9 10 11 12
| // the SetCookie function
function setCookie(cookiename, cookievalue, daysexpires) {
var cookieexpires = "";
if (daysexpires > 0) {
var today = new Date();
today.setTime(today.getTime() + (daysexpires * 864e5));
cookieexpires = "expires=" + today.toUTCString() + ";";
} else if (daysexpires < 0) {
cookieexpires = "expires=Thu, 1 Jan 1970 00:00:01 UTC;";
}
document.cookie = cookiename + "=" + cookievalue + ";" + cookieexpires + "path=/";
} |
Like I said, a simple function, applicable for most scenarios.
The function requires a Cookie name and value, and optionally the number of days before it expires.
Initially we create a new empty string for the expiration text.
If the number of days is greater than zero, we will create a new Date object and set it to the current date and time increased by the number of days expressed in milliseconds.
The strange 864e5 is the short scientific notation for 86400000 which is the outcome of:
hours in a day × minutes in an hour × seconds in a minute × milliseconds in a second =
24 × 60 × 60 × 1000 =
86,400,000 =
864e5
If the number of days is less than zero (-1 for example) then the expiration date is set to “Thu, 1 Jan 1970 00:00:01 UTC” which effectively deletes the cookie.
Using the Web Developer tools, you can verify that the expiration date and path are indeed set.
An example with some tests:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| // Set a few cookies (website)
setCookie("username1", "Hans1", 0); // session cookie
alert('Cookies set: ' + document.cookie);
setCookie("username1", "Hans1", -1); // delete cookie
alert('Cookies set: ' + document.cookie);
setCookie("username3", "Hans3", 7); // expires in a week
alert('Cookies set: ' + document.cookie);
// the SetCookie function
function setCookie(cookiename, cookievalue, daysexpires) {
var cookieexpires = "";
if (daysexpires > 0) {
var today = new Date();
today.setTime(today.getTime() + (daysexpires * 864e5));
cookieexpires = "expires=" + today.toUTCString() + ";";
} else if (daysexpires < 0) {
cookieexpires = "expires=Thu, 1 Jan 1970 00:00:01 UTC;";
}
document.cookie = cookiename + "=" + cookievalue + ";" + cookieexpires + "path=/";
} |
deleteAllCookies() – Clear and Remove all Cookies for this path
This function is also limited to a certain set of cookies, but again covers the majority. It takes one parameter: the path. If you leave it blank then it will take the root path (‘/’).
1 2 3 4 5 6
| // the DeleteAllCookies
function deleteAllCookies(cookiepath = '/') {
document.cookie.split(';').forEach(function(c) {
document.cookie = c.trim().split('=')[0] + '=;expires=Thu, 01 Jan 1970 00:00:01 UTC; path='+cookiepath;
});
} |
As you can see this function is a little bit more complex, but basically we define a function in the function, applies for each cookie we find (based on splitting the string on the semi colons), where we look for what is in front of the equal (=) sign and paste no value, expiration time and path.
An example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| // Set a few cookies (website)
setCookie("username1", "Hans1", 7); // session cookie
setCookie("username2", "Hans2", 14); // delete cookie
setCookie("username3", "Hans3", 365); // expires in a week
alert('Cookies set: ' + document.cookie);
deleteAllCookies();
alert('Cookies remaining: ' + document.cookie);
// the SetCookie function
function setCookie(cookiename, cookievalue, daysexpires) {
var cookieexpires = "";
if (daysexpires > 0) {
var today = new Date();
today.setTime(today.getTime() + (daysexpires * 864e5));
cookieexpires = "expires=" + today.toUTCString() + ";";
} else if (daysexpires < 0) {
cookieexpires = "expires=Thu, 1 Jan 1970 00:00:01 UTC;";
}
document.cookie = cookiename + "=" + cookievalue + ";" + cookieexpires + "path=/";
}
// the DeleteAllCookies
function deleteAllCookies(cookiepath = '/') {
document.cookie.split(';').forEach(function(c) {
document.cookie = c.trim().split('=')[0] + '=;expires=Thu, 01 Jan 1970 00:00:01 UTC; path='+cookiepath;
});
} |
All functions combined
Below all functions combined so you can easily copy and paste them for your own projects;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| // the getCookie function
function getCookie(cookiename) {
var name = cookiename + "=";
var arrayOfCookies = decodeURIComponent(document.cookie).split(';');
for (var i = 0; i < arrayOfCookies.length; i++) {
var aCookie = arrayOfCookies[i];
while (aCookie.charAt(0) == ' ') {
aCookie = aCookie.substring(1);
}
if (aCookie.indexOf(name) == 0) {
return aCookie.substring(name.length, aCookie.length);
}
}
return "";
}
// the SetCookie function (daysexpires <0 = delete, =0 = session cookie, >0 = days expire from today
function setCookie(cookiename, cookievalue, daysexpires) {
var cookieexpires = "";
if (daysexpires > 0) {
var today = new Date();
today.setTime(today.getTime() + (daysexpires * 864e5));
cookieexpires = "expires=" + today.toUTCString() + ";";
} else if (daysexpires < 0) {
cookieexpires = "expires=Thu, 1 Jan 1970 00:00:01 UTC;";
}
document.cookie = cookiename + "=" + cookievalue + ";" + cookieexpires + "path=/";
}
// the DeleteAllCookies
function deleteAllCookies(cookiepath = '/') {
document.cookie.split(';').forEach(function(c) {
document.cookie = c.trim().split('=')[0] + '=;expires=Thu, 01 Jan 1970 00:00:01 UTC; path='+cookiepath;
});
} |
Comments
There are no comments yet.
You can post your own comments by using the form below, or reply to existing comments by using the "Reply" button.